Copyright © Pixel Ellipsis
All Rights reserved
E-Mail: info@pixel-ellipsis.net
Wannabe game developers get quite scared when they see SED open for the very first time. I try to calm their fears by telling them that the script editor is nothing more than a beefed-up version of... Notepad.
Yeah, SED is nothing more than a text editor which has got some pretty features, with the goal of helping you write better code, and do it faster!
We will go through a simple program that creates a rotating spotlight, just like the ones used by the real guards to make sure that nobody is escaping their prison.
I will comment all the code lines thoroughly, so you won't miss a thing - it's a promise! But first, let's see the code in action:
Working with the script editor - SED
Looks quite good, eh? And trust me - it looks even better in motion!
Here's how the source code looks like:
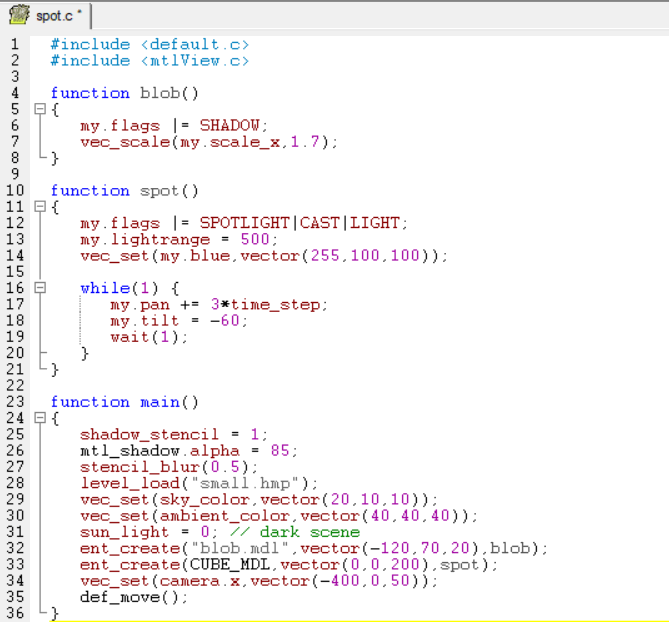
As you can see, we are getting a great light effect with less than 40 lines of code - a very impressive performance, if you ask me.
Let's go through the entire code, step by step:
#include <default.c>
#include <mtlView.c>
The two lines of code above are very similar. They include other built-in code libraries named default.c and mtlView.c, which contain useful functions. Gamestudio comes with a huge library of functions that can be used in our projects, helping us create impressive games without spending hundreds or thousands of hours coding them. It is one of its key efficiency secrets.
function blob()
{
my.flags |= SHADOW;
vec_scale(my.scale_x,1.7);
}
This function is attached to the blob entity that was placed in the level. The code that controls the blob is placed inside the curly brackets.
The first line tells the entity to display a shadow whenever it is illuminated by a light source. You will see the blob shadow if you take another look at the picture above.
The second line scales the entity, making it taller. By setting its scale_x to 1.7, the entity will become 70% taller, get it? A scale_x factor of 3 would make the entity 200% taller, and so on. We need to subtract 100 from the scaling factor, because the entity itself has a scale_x value of 100% (its normal height).
function spot()
{
my.flags |= SPOTLIGHT|CAST|LIGHT;
my.lightrange = 500;
vec_set(my.blue,vector(255,100,100));
while(1) {
my.pan += 3*time_step;
my.tilt = -60;
wait(1);
}
}
Function spot() is a bit more complex. The first line of code sets the SPOTLIGHT, CAST and LIGHT flags for the light source. By the way: you can find all the predefined flags and functions in the Gamestudio manual, which is installed in the same folder with the engine.
The next line of code sets the lightrange to 500 quants, and the one that follows it sets the light color to a slightly blueish one. The colors are listed in the BGR order, so this time we've gotten a value of 255 for blue, 100 for green and 100 for red.
The while(1) loop is executed at all times, so the 3 lines of code inside it won't stop until the engine is shut down.
The first one rotates the light around, the other one sets its tilt angle to -60 degrees, making it point downwards, and the third one tells the engine to wait for a frame. Without this wait instruction, all the CPU resources would be used by that while loop, and your computer would freeze.
function main()
{
shadow_stencil = 1;
mtl_shadow.alpha = 85;
stencil_blur(0.5);
level_load("small.hmp");
vec_set(sky_color,vector(20,10,10));
vec_set(ambient_color,vector(40,40,40));
sun_light = 0; // dark scene
ent_create("blob.mdl",vector(-120,70,20),blob);
ent_create(CUBE_MDL,vector(0,0,200),spot);
vec_set(camera.x,vector(-400,0,50));
def_move();
}
Function main must exist in each Gamestudio application, because it is executed automatically every time you run the engine. It sets two parameters for the shadows, which will make them look better, and then it calls a predefined function - stencil_blur(0.5), a part of the mtlView.c library which was included at the beginning of our code.
The following lines set the sky color and ambient color; the same BGR rule applies here as well. By setting a sun_light value of zero, we create a dark scene, which emphasizes our moving light.
The two ent_create lines of code create our blob and a cube that will be the origin of our light spot. Finaly, the vec_set(camera.x...) line of code sets the initial camera (viewing) position.
The last line of code calls a function from one of the included libraries - default.c. It's a simple, elegant code movement snippet which allow us to move the camera around.
This includes our SED tutorial; I hope that you like it. If everything goes as planned, the next tutorial I'm going to create will discuss a simple multiplayer game, for people like you and me who simply love 3D shooters. Stay tuned!